Guard Class Template Reference
#include <Guard.h>
Inheritance diagram for Guard:
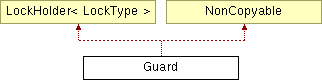
Public Member Functions | |
Guard (LockType &lock) | |
Guard (LockType &lock, unsigned long timeout) | |
template<class U, class V> | |
Guard (Guard< U, V > &g) | |
Guard (Guard &g) | |
template<class U, class V> | |
Guard (Guard< U, V > &g, LockType &lock) | |
Guard (Guard &g, LockType &lock) | |
~Guard () throw () | |
Friends | |
class | LockHolder<LockType> |
Detailed Description
template<class LockType, class LockingPolicy = LockedScope>
class ZThread::Guard< LockType, LockingPolicy >
- Author:
- Eric Crahen <http://www.code-foo.com>
- Date:
- <2003-07-16T17:55:42-0400>
- Version:
- 2.2.0
For instance, consider a case in which a class or program have a Mutex object associated with it. Access can be serialized with a Guard as shown below.
Mutex _mtx;
void guarded() {
Guard<Mutex> g(_mtx);
}
The Guard will lock the synchronization object when it is created and automatically unlock it when it goes out of scope. This eliminates common mistakes like forgetting to unlock your mutex.
An alternative to the above example would be
void guarded() {
(Guard<Mutex>)(_mtx);
}
HOWEVER; using a Guard in this method is dangerous. Depending on your compiler an anonymous variable like this can go out of scope immediately which can result in unexpected behavior. - This is the case with MSVC and was the reason for introducing assertions into the Win32_MutexImpl to track this problem down
Constructor & Destructor Documentation
|
Create a Guard that enforces a the effective protection scope throughout the lifetime of the Guard object or until the protection scope is modified by another Guard.
|
|
Create a Guard that enforces a the effective protection scope throughout the lifetime of the Guard object or until the protection scope is modified by another Guard.
|
|
Create a Guard that shares the effective protection scope from the given Guard to this Guard.
|
|
Create a Guard that shares the effective protection scope from the given Guard to this Guard.
|
|
Create a Guard that transfers the effective protection scope from the given Guard to this Guard.
|
|
Create a Guard that transfers the effective protection scope from the given Guard to this Guard.
|
|
Unlock a given Lockable object with the destruction of this Guard |
The documentation for this class was generated from the following file:
- Guard.h