ThreadLocal Class Template Reference
#include <ThreadLocal.h>
Inheritance diagram for ThreadLocal:
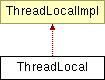
Public Member Functions | |
T | get () const |
void | set (T v) const |
void | clear () const |
Static Public Member Functions | |
void | clearAll () |
Detailed Description
template<typename T, typename InitialValueT = ThreadLocalImpl::InitialValueFn<T>, typename ChildValueT = ThreadLocalImpl::UniqueChildValueFn, typename InheritableValueT = ThreadLocalImpl::InheritableValueFn>
class ZThread::ThreadLocal< T, InitialValueT, ChildValueT, InheritableValueT >
- Author:
- Eric Crahen <http://www.code-foo.com>
- Date:
- <2003-07-27T11:18:21-0400>
- Version:
- 2.3.0
The first time a thread accesses the value associated with a thread local storage context, a value is created. That value is either an initial value (determined by InitialValueT) or an inherited value (determined by ChildValueT).
- If a threads parent had no value associated with a ThreadLocal when the thread was created, then the InitialValueT functor is used to create an initial value.
- If a threads parent did have a value associated with a ThreadLocal when the thread was created, then the childValueT functor is used to create an initial value.
Not all ThreadLocal's support the inheritance of values from parent threads. The default behavoir is to create values through the InitialValueT functor for all thread when they first access a thread local storage context.
- Inheritance is enabled automatically when a user supplies a ChildValueT functor other than the default one supplied.
- Inheritance can be controlled explicitly by the user through a third functor, InheritableValueT.
Examples
Default initial value
A ThreadLocal that does not inherit, and uses the default value for an int as its initial value.
#include "zthread/ThreadLocal.h" #include "zthread/Thread.h" #include <iostream> using namespace ZThread; class aRunnable : public Runnable { ThreadLocal<int> localValue; public: void run() { std::cout << localValue.get() << std::endl; } }; int main() { // Create a shared task to display ThreadLocal values Task task(new aRunnable); Thread t0(task); t0.wait(); Thread t1(task); t1.wait(); Thread t2(task); t2.wait(); // Output: // 0 // 0 // 0 return 0; }
User-specified initial value
A ThreadLocal that does not inherit, and uses a custom initial value.
#include "zthread/ThreadLocal.h" #include "zthread/Thread.h" #include <iostream> using namespace ZThread; struct anInitialValueFn { int operator()() { static int next = 100; int val = next; next += 100; return val; } }; class aRunnable : public Runnable { ThreadLocal<int, anInitialValueFn> localValue; public: void run() { std::cout << localValue.get() << std::endl; } }; int main() { // Create a shared task to display ThreadLocal values Task task(new aRunnable); Thread t0(task); t0.wait(); Thread t1(task); t1.wait(); Thread t2(task); t2.wait(); // Output: // 100 // 200 // 300 return 0; }
User-specified inherited value
A ThreadLocal that does inherit and modify child values. (The default initial value functor is used)
#include "zthread/ThreadLocal.h" #include "zthread/Thread.h" #include <iostream> using namespace ZThread; struct anInheritedValueFn { int operator()(int val) { return val + 100; } }; // This Runnable associates no ThreadLocal value in the main thread; so // none of the child threads have anything to inherit. class aRunnable : public Runnable { ThreadLocal<int, ThreadLocalImpl::InitialValueFn<int>, anInheritedValueFn> localValue; public: void run() { std::cout << localValue.get() << std::endl; } }; // This Runnable associates a ThreadLocal value in the main thread which // is inherited when the child threads are created. class anotherRunnable : public Runnable { ThreadLocal<int, ThreadLocalImpl::InitialValueFn<int>, anInheritedValueFn> localValue; public: anotherRunnable() { localValue.set(100); } void run() { std::cout << localValue.get() << std::endl; } }; int main() { // Create a shared task to display ThreadLocal values Task task(new aRunnable); Thread t0(task); t0.wait(); Thread t1(task); t1.wait(); Thread t2(task); t2.wait(); // Output: // 0 // 0 // 0 task = Task(new anotherRunnable); Thread t10(task); t10.wait(); Thread t11(task); t11.wait(); Thread t12(task); t12.wait(); // Output: // 200 // 200 // 200 return 0; }
Parameters
InitialValueT
This template parameter should indicate the functor used to set the initial value. It should support the following operator:
// required operator T operator()
// supported expression InitialValueT()()
ChildValueT
This template parameter should indicate the functor used to set the value that will be inherited by thread whose parent have associated a value with the ThreadLocal's context at the time they are created. It should support the following operator:
// required operator T operator(const T& parentValue)
// supported expression ChildValueT()(parentValue)
InheritableValueT
This template parameter should indicate the functor, used to determine wheather or not this ThreadLocal will allow values from a parent threads context to be inherited by child threads when they are created. It should support the following operator:
// required operator bool operator(const T& childValueFunctor)
// supported expression InheritableValueT()( ChildValueT() )
Member Function Documentation
|
Remove any value current associated with this ThreadLocal.
Reimplemented from ThreadLocalImpl. |
|
Remove any value current associated with any ThreadLocal.
Reimplemented from ThreadLocalImpl. |
|
Get the value associated with the context (this ThreadLocal and the calling thread) of the invoker. If no value is currently associated, then an intial value is created and associated; that value is returned.
|
|
Replace the value associated with the context (this ThreadLocal and the calling thread) of the invoker. If no value is currently associated, then an intial value is first created and subsequently replaced by the new value.
|
The documentation for this class was generated from the following file:
- ThreadLocal.h